Drupal 8 has a great Plugin API that not only allows one to write plugins that modify Drupal core’s behavior, but also to define your own pluggable system that you and others can plug into as well. The Plugin API documentation has a number of examples that cover many common use cases for plugins and configuration entities, but none quite covered my use case, so hopefully this article can help fill some of the gaps. I’m going to use an actual example that I built to help ground the discussion of abstract concepts in something more concrete — the abstractions like Annotations, EntityWithPluginCollectionInterface
, PluginFormInterface
, Derivatives, and others are powerful, but it can be tough to pin down how and when to actually use one versus another.
The Challenge
My end goal is a module that creates Drupal nodes of a custom “Event” content-type based on a variety of external calendaring systems. My campus uses the Resource25 calendaring system which will be the source of most events, while others may be sourced from Outlook calendars, Google Calendars, or other data sources. What the source systems are and how they are connected to will never be completely unified and will change over time, so I want to create a flexible, pluggable, system that will allow new plugins to be written that just cover how to fetch from new source systems without reinventing the whole thing every time we need to add a data source.
There are two complications that lift this implementation out of the realm covered in a straight-forward manner in the Drupal documentation:
- Each plugin has both standard configuration options (such as “Enabled”) as well as plugin-specific configuration options.
- For a given Drupal site, there might be multiple instances of each source plugin that are configured to pull from different calendar feeds using the same protocols and methods.
This system is very similar to Drupal-core’s Block system. The Block system defines an API for “Block Plugins” where each block-plugin has PHP code that drives it. Multiple instances of each block-plugin can be placed in the page, each with their own settings. For example, the System Menu Block Plugin in the system module can have multiple instances added to the block-layout, each configured to display a different menu with different settings for initial menu-level and depth of menu-levels visible as well as the standard settings for title and display of the title.
In fact, the Plugin API documentation specifically recommends using the Block system as an example. Unfortunately this is a complicated example to follow because the Block system is scattered between Drupal-core library files and the Block module, rather than all contained in one place.
What is needed?
I’m going to skip over the basics of the module skeleton as these are well documented already in order to focus on the parts that are needed to achieve the system described above.
Here are the pieces that we need to get functioning and which I will dive further into:
- Defining the plugins themselves with a Plugin Manager and discovery mechanisms so that Drupal knows what plugins are available.
- Defining the Configuration Entity for our plugin system so that instances can be configured and exported along with the rest of our site configuration.
- Defining dynamic links so that we can create new instances for each plugin installed.
- Allowing plugins to define extra configuration fields/properties that are particular to their needs.
A snapshot of the resulting module is available on GitHub at adamfranco/middlebury_event_sync. I’ll be linking to code in the module rather than copying it here.
Defining the Plugins
For this system, we are going to define a single Plugin Manager that will provide discovery and loading of our Event Source plugins and a Plugin Interface that all of our Event Source plugins will have to implement.
The Plugin Manager gets a listing in our module.services.yml
as well as a class that defines what the alter-hooks are and what the plugin name-space is. More on Services and Dependency Injection can be found on drupal.org.
Next up is an Annotation class at src/AnnotationEventSource.php
which defines the parameters that will be used in each plugin class‘s doc-block to identify that plugin class as one of our plugins. This is the key to the discovery mechanism we’re using — the annotation in the doc-block registers the plugin so that it is available through our Plugin Manager.
We also define Plugin Interface which defines common methods for all of our Event Source plugins. This isn’t strictly required for all plugin systems as the PluginInspectionInterface
and/or ConfigurablePluginInterface
can be implemented by plugins directly, but I wanted all plugins to implement a few common methods for standard settings and actual operations.
The manager, service, annotation, and plugin interface are the things we need to define to allow our plugins in src/Plugin/EventSource/
to be discovered and available to Drupal. Now that we have defined our plugins, we can use \Drupal::service('plugin.manager.event_source')->getDefinitions()
to access all of the Event Source plugins installed in our site, both those provided by our module as well as those provided by other modules.
Configuration Entities
The next task is to define a configuration entity, the instances of which will hold the configuration for each service we are connecting to and reference the plugin that will be used to do the connecting. Configuration in Drupal 8 is a great new system that allows configuration to be modified in a development environment, exported, version-controlled, and then imported in a production environment. Configuration Entities allow units of configuration to be created, listed, edited, and deleted like other entities in Drupal (nodes, users, taxonomy-terms, menu items, etc). For this module, I need to use config entities because we don’t have just a single global setting, but rather zero or more configuration sets for each plugin.
Our config entity is a single class that extends ConfigEntityBase
and has a number of annotations that point to the Controllers and Forms used to list and edit the instances of the Entity. Our config entity class also implements an interface I created that defines how to access the plugin associated with the config entity, not something available by default.
If we just wanted to have a single config entity for each plugin, this would all be enough. However we want to be able to have multiple config entity instances associated with each plugin. To achieve this our config entity must also implement the confusingly named EntityWithPluginCollectionInterface
which provides access an EventSourcePluginCollection
that we define. This “Plugin Collection” handles the mapping between config entity instances and the plugin instances that house our actual data-fetching code. Via this Plugin Collection we can pass a config entity (or its settings) off to the plugin instance so that the plugin instance has access to data in addition to its methods.
After defining the config entity and getting it working, it is a good idea to define a Configuration Schema in yml. The primary purpose of this is to allow translation of your configuration — it doesn’t seem to affect the actual export of the configuration.
Our config entity is pretty light-weight — it stores its id (a.k.a. machine-name), label, which plugin-id it is associated with, which module is providing that plugin, and finally an arbitrary set of plugin-settings defined by the plugin. These plugin settings include some common ones such as enabled, ttl, and time-shift, as well as plugin-specific settings like URIs, username, and password. There are other ways that the plugin settings could be injected into the config entity, but I felt that putting them in a single container called “settings” was flexible and simple and avoided potential conflicts between the keys needed by the config entity and the plugin.
Dynamic Links to add instances
A missing piece with what we have so far are the links to create new config entity instances. Because new plugins may be provided by other installed modules, we can’t hard-code our links to add new instances in our action links. Instead we need to implement a Derivative plugin that generates a list of links based on the available plugins and point to this Deriver from our module’s module.links.action.yml
.
Extra configuration fields per plugin
To assist with common settings and functionality, I created an abstract base class that all of my plugins can inherit. This base class implements the PluginFormInterface
to provide form fields on the config entity’s editing screens. Each plugin can override these methods to provide and handle additional fields while leaving the common fields to the base class. These fields are handled as a sub-form loaded in our config-entity form class.
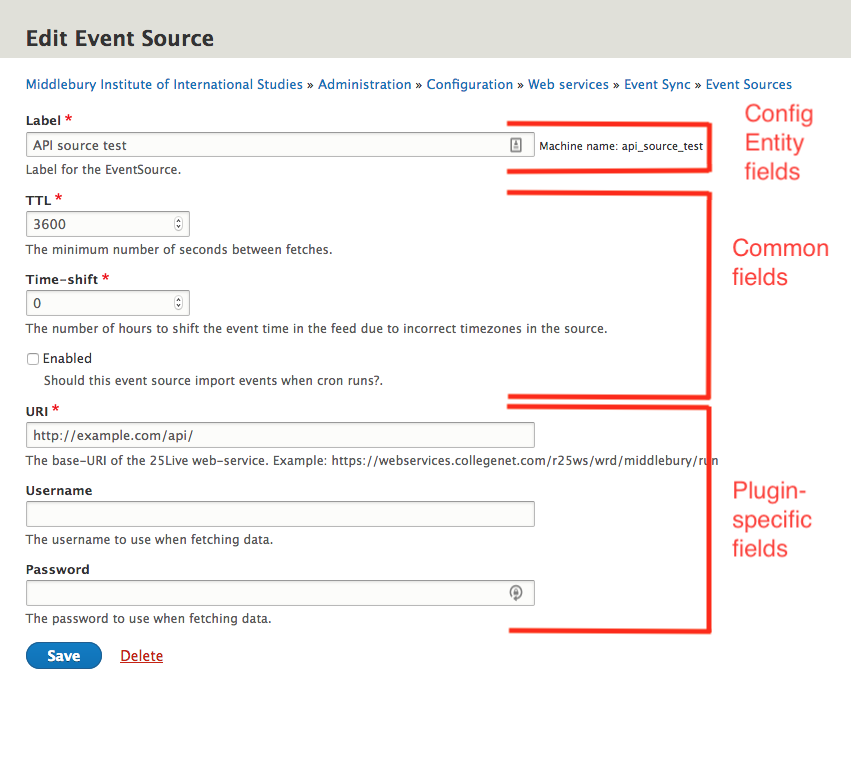
Event Source editing form showing fields provided by the configuration entity itself (label & machine name), common fields from our abstract base class (TTL, time-shift, enabled) and plugin-specific fields (URI, username, password).
The Result
Once the items above were in place I now have a system that lets me create as many instances of each plugin as needed. The buttons to add a new instance are provided by the Deriver and the listing itself by a controller.
Hopefully this overview can point you enough in the right direction to implement your own Drupal plugin systems for other purposes.
Resources
Below are links to the documentation and resources that I used in writing this pluggable system and this article:
- Plugin API documentation on Drupal.org
- Plugin API on api.drupal.org
- Creating a configuration entity type in Drupal 8
- Principles of Configuration Management – Part Three
- Drupal 8 Print Module Port: The Plugin API (1/5)
- Dynamic menu links in Drupal 8 with plugin derivatives
- Module snapshot on GitHub: adamfranco/middlebury_event_sync